-
Notifications
You must be signed in to change notification settings - Fork 0
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
算法整理 #13
Comments
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
时间复杂度
评估执行程序所需的时间。可以估算出程序对处理器的使用程度。
每个算法中语句执行的次数叫做时间频度,叫T(n)
O(f(n))=时间复杂度。
O(f(n)中的f(n)的值可以为1、n、logn、n²等,因此我们可以将O(1)、O(n)、O(logn)、O(n²)分别可以称为常数阶、线性阶、对数阶和平方阶
计算法则:
1.用常数1来取代运行时间中所有加法常数。
2.修改后的运行次数函数中,只保留最高阶项
3.如果最高阶项存在且不是1,则去除与这个项相乘的常数。
常用的时间复杂度按照耗费的时间从小到大依次是:
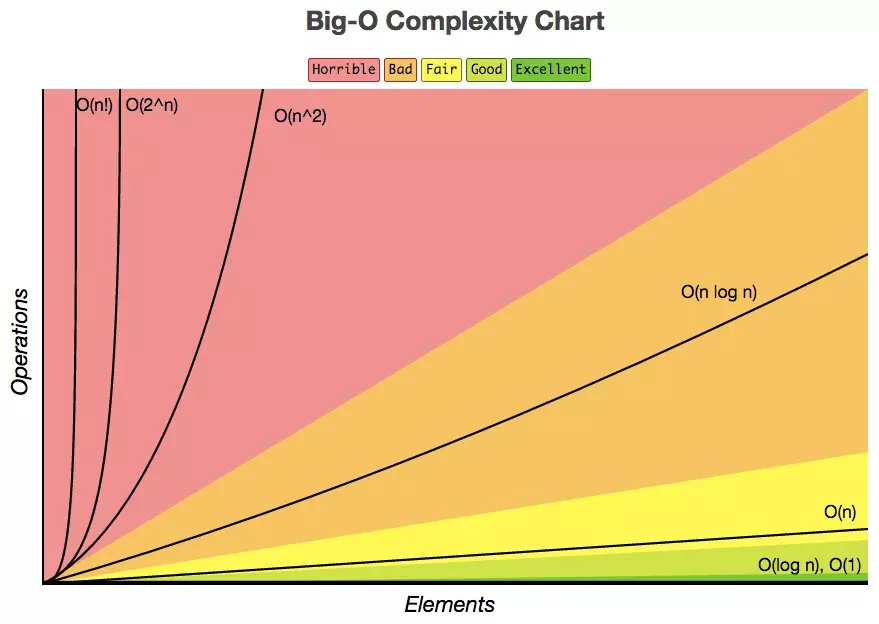
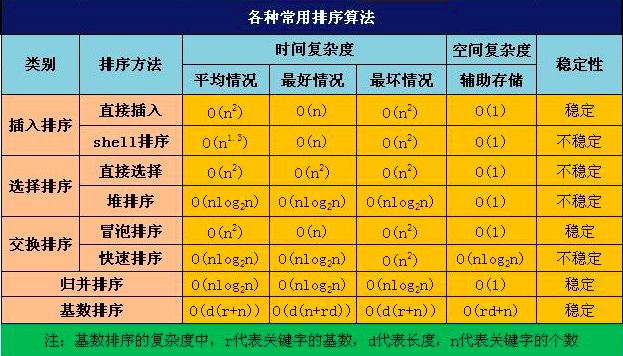
O(1)<O(logn)<O(n)<O(nlogn)<O(n²)<O(n³)<O(2ⁿ)<O(n!)
常用算法时间复杂度:
排序
2.冒泡排序
The text was updated successfully, but these errors were encountered: